Need help in Java: How to match two pictures?
4 posters
Page 1 of 1
Need help in Java: How to match two pictures?
I have used gridLayout to make some buttons. In those buttons I have used ImageIcon and setIcon(icon) method to set image for that buttons.
Now how will match 2 pictures if those 2 pictures are same? I have use used icon1.equals(icon2) method but it is not working.
Now how will match 2 pictures if those 2 pictures are same? I have use used icon1.equals(icon2) method but it is not working.
Last edited by LordAmit on Thu Oct 28, 2010 9:11 pm; edited 1 time in total (Reason for editing : Use icons, and use the programming language name in topic title please)
bit0223-sujon- Global Moderator-RC
- Course(s) :
- BIT
Posts : 98
Points : 218
Re: Need help in Java: How to match two pictures?
Sujon, please use the appropriate icon of topic when you are posting in the programming zone 
Also, use the keyword Java in your topic title, so that we can find it when we use the search functionality of this forum.
Now, helping you...
Instead of giving you a code that should help you, lets check the definition of equals method.
you should use button.getIcon() method instead, which returns the image it is holding.
Here is the explanatory code. I am posting the same code twice. Please tell me which one looks better.
version 1:
version 2:

Also, use the keyword Java in your topic title, so that we can find it when we use the search functionality of this forum.
Now, helping you...
Instead of giving you a code that should help you, lets check the definition of equals method.
As you can see, icon1 and icon2 are not same objects. Which is why it is saying that they are not same.JavaDoc wrote:
The equals method for class Object implements the most discriminating possible equivalence relation on objects; that is, for any non-null reference values x and y, this method returns true if and only if x and y refer to the same object (x == y has the value true).
you should use button.getIcon() method instead, which returns the image it is holding.
Here is the explanatory code. I am posting the same code twice. Please tell me which one looks better.
version 1:
- Code:
ImageIcon icon1 = new ImageIcon("src/halum/lesson.gif");
ImageIcon icon2 = new ImageIcon("src/halum/lesson1-1.jpg");
JButton button1 = new JButton(icon1);
JButton button2 = new JButton(icon2);
JButton button3 = new JButton(icon1);
if(icon1.equals(icon2)) {
System.out.println("This line ain't gonna be shown dude. Because...");
}else {
System.out.println("They ain't same object you know :| ");
}
if (button1.getIcon()==button2.getIcon()) {
System.out.println("This is a better method. but They ain't same.");
}else {
System.out.println("Not gonna be printed");
}
if(button1.getIcon() == button3.getIcon()) {
System.out.println("this is true");
System.out.println("So this is what you should use");
}else {
System.out.println("Do we really need this else?");
}
if (button1.getIcon().equals(button3.getIcon())) {
System.out.println("This should work as well. As both buttons use same image icon");
}
version 2:
Re: Need help in Java: How to match two pictures?
As far as I know, when you are checking whether the values of two objects are same then you should use equals() function, if you are checking whether two objects are holding same reference then you should use "=="
Using button1.getIcon() you can get the Icon of the button. Now we need the content of the icon or value inside the icon. If you are comparing the to images by their name(the parameter inside ImageIcon object) you can use toString() to compare.
As you are checking whether the icons of the two buttons is same, first you should get the icon of the buttons and then check it.bit0223-sujon wrote:I have use used icon1.equals(icon2) method but it is not working.
Using button1.getIcon() you can get the Icon of the button. Now we need the content of the icon or value inside the icon. If you are comparing the to images by their name(the parameter inside ImageIcon object) you can use toString() to compare.
- Code:
button1.getIcon().toString().equals(button2.getIcon().toString())
Last edited by BIT0107-toma on Fri Oct 29, 2010 4:34 am; edited 1 time in total (Reason for editing : correction of information)
BIT0107-Toma- Programmer
- Course(s) :
- BIT
Blood Group : B+
Posts : 280
Points : 453
Re: Need help in Java: How to match two pictures?
A very nice method explained by Toma
And effective as well.
BTW
After going through 6 websites, and then experimenting myself, I think I should say that there is a slight problem in your statement.
When you are referring to objects, unless overridden, the equals method actually compares the references of them. So, it is the same as "==".
Here is what javadoc says:
But this is not applicable for String type.
As javadoc says,
I hope you will not misunderstand me
I am not trying to criticize you, but rather sharing what I just learned after reading your statement and thinking about it. And for giving me opportunity of thinking, I thank you.
Here is the code I used for experimenting:

BTW

As far as I know, when you are checking whether the values of two objects are same then you should use equals() function
After going through 6 websites, and then experimenting myself, I think I should say that there is a slight problem in your statement.
When you are referring to objects, unless overridden, the equals method actually compares the references of them. So, it is the same as "==".
Here is what javadoc says:
The equals method for class Object implements the most discriminating possible equivalence relation on objects; that is, for any non-null reference values x and y, this method returns true if and only if x and y refer to the same object (x == y has the value true).
But this is not applicable for String type.
As javadoc says,
The result is true if and only if the argument is not null and is a String object that represents the same sequence of characters as this object.
I hope you will not misunderstand me

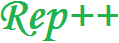
Here is the code I used for experimenting:
- Code:
package halum;
public class Testing {
private int a;
public Testing(int b) {
// TODO Auto-generated constructor stub
a=b;
}
public static void main(String[] args) {
Testing testing = new Testing(1);
Testing testing2 = testing;
Testing testing3 = new Testing(1);
System.out.println("Object Part");
System.out.println(testing == testing2);
System.out.println(testing == testing3);
System.out.println(testing.equals(testing2));
System.out.println(testing.equals(testing3));
System.out.println("String part");
String aString = "Hi";
String bString = aString;
String cString = "Hi";
System.out.println(aString == bString);
System.out.println(aString == cString);
System.out.println(aString.equals(bString));
System.out.println(aString.equals(cString));
System.out.println(aString.compareTo(bString));
System.out.println(aString.compareTo(cString));
}
}
Re: Need help in Java: How to match two pictures?
I am not telling that you are completely wrong. But I will request you to read this.BIT0122-Amit wrote:When you are referring to objects, unless overridden, the equals method actually compares the references of them. So, it is the same as "==".
It says:
Here, "it" means "equals()" function.It has been defined in a meaningful way for most Java core classes. If it's not defined for a (user) class, it behaves the same as ==.
It also says:
the equals() method makes a == test first, it can be fairly fast when the objects are identical. It only compares the values if the two references are not identical.
BIT0107-Toma- Programmer
- Course(s) :
- BIT
Blood Group : B+
Posts : 280
Points : 453
Re: Need help in Java: How to match two pictures?
That is one of the 6 pages I went through last night 
If I am not totally wrong, then I am partially wrong according to you. And the correction you referred is,
Perhaps you missed this (now bold) part though:
Overridden, user-defined... I can not really see the difference.
Anyway, I only posted that because
Again, we were here considering ImageIcon objects. so, if we invoke the equals method on them directly, how are they going to compare them? What are the values?
By size? By name? Both of the combination? or something else?
which is why, ImageIcon only inherited the "==" comparison in equals() method.

If I am not totally wrong, then I am partially wrong according to you. And the correction you referred is,
It has been defined in a meaningful way for most Java core classes. If it's not defined for a (user) class, it behaves the same as ==.
Perhaps you missed this (now bold) part though:
When you are referring to objects, unless overridden, the equals method actually compares the references of them. So, it is the same as "==".
Overridden, user-defined... I can not really see the difference.
Anyway, I only posted that because
where you didn't mention the exception.
when you are checking whether the values of two objects are same then you should use equals() function,
Again, we were here considering ImageIcon objects. so, if we invoke the equals method on them directly, how are they going to compare them? What are the values?
By size? By name? Both of the combination? or something else?
which is why, ImageIcon only inherited the "==" comparison in equals() method.
Re: Need help in Java: How to match two pictures?
That's my point. That's why I did not wrote "button1.getIcon().equals(button2.getIcon())"BIT0122-Amit wrote:Again, we were here considering ImageIcon objects. so, if we invoke the equals method on them directly, how are they going to compare them? What are the values?
By size? By name? Both of the combination? or something else?
If Sujon is using two different object with same image then "==" is not going to work and the button.getIcon().toString() function returns the name of the images and compares their value
BIT0107-Toma- Programmer
- Course(s) :
- BIT
Blood Group : B+
Posts : 280
Points : 453
Re: Need help in Java: How to match two pictures?
BIT0107-toma wrote:
That's my point. That's why I did not wrote "button1.getIcon().equals(button2.getIcon())"
If Sujon is using two different object with same image then "==" is not going to work and the button.getIcon().toString() function returns the name of the images and compares their value
That's where you got it wrong there

When you are using the getIcon method, it returns the icon object which was used in the Icon holding object. (which is, in this case, a JButton)
So, if you use the same icon object in two buttons, and then use
- Code:
button1.getIcon() == button2.getIcon()
It will work.
Example?
- Code:
ImageIcon icon1 = new ImageIcon("src/halum/lesson1-0.jpg");
ImageIcon icon2 = new ImageIcon("src/halum/lesson1-1.jpg");
JButton button1 = new JButton(icon1);
JButton button2 = new JButton(icon2);
JButton button3 = new JButton(icon1);
if(icon1.equals(icon2)) {
System.out.println("This line ain't gonna be shown dude. Because...");
}else {
System.out.println("They ain't same object you know :| ");
}
if (button1.getIcon()==button2.getIcon()) {
System.out.println("This is a better method. but They ain't same.");
}else {
System.out.println("Not gonna be printed");
}
if(button1.getIcon() == button3.getIcon()) {
System.out.println("this is true");
System.out.println("So this is what you should use");
}else {
System.out.println("Do we really need this else?");
}
if (button1.getIcon().equals(button3.getIcon())) {
System.out.println("This should work as well. As both buttons use same image icon");
}
Edit:
I think I mentioned that your method in your first reply was a nice one.
Re: Need help in Java: How to match two pictures?
oi pula duita image kemne match korte hoi, dekhai dilam na?
Re: Need help in Java: How to match two pictures?
bit0112-rokon wrote:oi pula duita image kemne match korte hoi, dekhai dilam na?
একটু কষ্ট করে বাংলাটা বাংলায় লিখুন।

Re: Need help in Java: How to match two pictures?
@ Amit vaia & Toma apu-- A lot of thanks for helping me. Both this topic helped me to solve the problem.
@ Amit vaia-- at the 1st post the code you written in 2 formats- the 2nd one looks better and more comfrotable.
@ Rokon vaia -Yes the problem is now solved
@ Amit vaia-- at the 1st post the code you written in 2 formats- the 2nd one looks better and more comfrotable.
@ Rokon vaia -Yes the problem is now solved

bit0223-sujon- Global Moderator-RC
- Course(s) :
- BIT
Posts : 98
Points : 218
Re: Need help in Java: How to match two pictures?
Can you kindly change the topic icon from java to solved? It will help people understand that this problem is solved 
Also, do not forget to rep++ when you want to thank someone
It encourages to help even more!
It is easy :p Just press the + button.
And thanks for providing the feedback. It is good to know that the second format looks better.

Also, do not forget to rep++ when you want to thank someone

It is easy :p Just press the + button.
And thanks for providing the feedback. It is good to know that the second format looks better.
Page 1 of 1
Permissions in this forum:
You cannot reply to topics in this forum
|
|
» Cisco EHWIC SFP/GE WAN Card
» Huawei S1700-28GFR-4P-AC Price
» teach yourself C++ / Herbert Schildt Solutions
» teach yourself c by herbert schildt pdf
» ASA 5506X With Firepower ASA5506-K9
» New Trends in Deal Business
» PoE Power Allocation for WS-C2960S-24PS-L
» How to cure back pain