Java Tutorial: Painting in swing components, part 1
4 posters
Page 1 of 1
Java Tutorial: Painting in swing components, part 1
Precaution: This tutorial will help you to know WHAT to do for painting on swing components. I have no intention to explain WHY to do what. Because I don't thing I can explain them all.
I am directly going to an example. Everything will be explained through comments.
Ok, here is another example with animation. Almost the same as the previous one. Just a bit of animations are added.
Please go through the program before running. I hope you'll have fun then.
and the output of the program

Now you can run the program, see what we've made.
Read part 2 (Interactive painting) of this tutorial.
I am directly going to an example. Everything will be explained through comments.
- Code:
import javax.swing.JPanel;
import javax.swing.JFrame;
import java.awt.Graphics;
/**
* We are inheriting the swing component JPanel to paint on
* it. We will draw some straight lines centering a point.
*/
public class PaintPanel extends JPanel {
// The radius of the circle. ie, the lenght of the
// lines.
private int r;
// The radius is passed through the constructor.
public PaintPanel(int r) {
this.r = r;
}
/**
* Overridding the paintComponent() method to paint. It
* is recommended that all paintings should be done in
* this method, not through in some other user defined
* functions. If you do so, do it on your own risk. You
* will have to handle some exceptions.
*
* @param g
* : Graphics assosiated with the panel
*/
protected void paintComponent(Graphics g) {
// You must call the super class method.
// Otherwise, you will see something unexpected
super.paintComponent(g);
/**
* Translating centre of painting coordinate to the
* centre of the panel. Default is the left top
* corner. I personally prefer (also should you)
* shifting operators if possible than
* multiplication or division. Shifting is much
* faster than arithematic operations.
*/
g.translate(getWidth() >> 1, getHeight() >> 1);
double theta = 0; // Holds the angular displacement
// of current line.
double fullCircle = Math.PI * 2; // The angle of a
// complete
// circle.
double increaseBy = Math.PI / 30; // Increase by 6
// degree (1
// second).
while (theta <= fullCircle) {
/*
* Math.PI*2 could be used instead of an extra
* variable. But but a double multiplication in
* each itteration of the loop is too much time
* consuming.
*/
int x = (int) (r * Math.cos(theta));
int y = (int) (r * Math.sin(theta));
g.drawLine(0, 0, x, y); // Drawing a line
theta += increaseBy; // Increasing the angular
// displacement
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("Paint Panel Test");
frame.setSize(500, 400);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new PaintPanel(100));
frame.setVisible(true);
}
}
Ok, here is another example with animation. Almost the same as the previous one. Just a bit of animations are added.
Please go through the program before running. I hope you'll have fun then.
and the output of the program
[click on the image to get it larger]

- Code:
// Thread classes used in this example are available in java.lang package. So
// you need not import them.
/**
* We are inheriting the swing component JPanel to paint on
* it. We will draw a straight line circulating around a
* point.
*/
class PaintPanelAnimated extends JPanel {
/**
* Some local variables in the privious example are
* declared as fields here. You will see why.
*/
private int r;
private double theta;
/**
* theta: We will not use a loop inside this class in
* this example, rather call paintComponent() several
* times from outside. So, this variable is made a
* field.
*/
private final double increaseBy, fullCircle;
/**
* final because we will never need to change them.
* Better if you say "we SHOULD NOT change them."
*/
public PaintPanelAnimated(int r) {
this.r = r;
theta = -Math.PI / 2; // start from here, not zero.
// You will see why...
increaseBy = Math.PI / 30; // 1 second
fullCircle = Math.PI * 2; // 360 degree
}
protected void paintComponent(Graphics g) {
super.paintComponent(g);
g.translate(getWidth() >> 1, getHeight() >> 1);
int x = (int) (r * Math.cos(theta));
int y = (int) (r * Math.sin(theta));
g.drawLine(0, 0, x, y);
theta += increaseBy;
}
public static void main(String[] args) {
JFrame frame = new JFrame("Paint Panel Test");
frame.setSize(500, 400);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
PaintPanelAnimate ppa = new PaintPanelAnimate(100);
frame.add(ppa);
frame.setVisible(true);
for (int i = 0; i < 20; i++) { // 20 has no
// significance at
// all.
ppa.repaint();
/**
* This is strongly recommended that you DO NOT
* call paintComponent() derectly. Neither from
* the code inside (except some cases, may be we
* will discuss it some other day), nor from
* outside (no exceptions here). To call
* paintComponent(), call repaint(). There are
* some intermediate method calls between
* repaint() and paintComponent(). I am skipping
* that part.
*/
try {
Thread.sleep(1000); // Waiting for 1 second
// (1000 miliseconds)
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
Now you can run the program, see what we've made.

Read part 2 (Interactive painting) of this tutorial.
Last edited by BIT0102-Mohaimin on Tue Feb 15, 2011 10:04 pm; edited 1 time in total (Reason for editing : Some comments in the codes were in inappropriate place)
BIT0102-Mohaimin- Programmer
- Course(s) :
- BIT
Blood Group : B+
Posts : 415
Points : 715
Re: Java Tutorial: Painting in swing components, part 1
Thanks a lot Mohaimin 
Circle with lines by mathematical calculations
I think, it will help me to learn easily to draw

Circle with lines by mathematical calculations

I think, it will help me to learn easily to draw

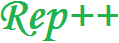
Re: Java Tutorial: Painting in swing components, part 1
Thanks Mohainin vai and
But I couldn't understand the function of the right shifting, why have you shifted that?
Would you explain it please?
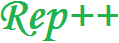
But I couldn't understand the function of the right shifting, why have you shifted that?
Would you explain it please?
BIT0208-Shuvo- Global Moderator-RC
- Course(s) :
- BIT
Blood Group : B+
Posts : 87
Points : 124
Re: Java Tutorial: Painting in swing components, part 1
You have read Shifting of binary numbers in EEE course right? Its the same thing happening here. Left shift means, all bits are shifted left, result is the same when you multiply by 2. For example.
If you want to multiply with 4, 8, 16 etc write
In the same way, shifting right means dividing by a power of 2
That means
Is it clear Or I should explain more?
- Code:
9 * 2
-> 1001 * 10
= 1001 << 1
= 10010
If you want to multiply with 4, 8, 16 etc write
- Code:
9 << 2
9 << 3
9 << 4
In the same way, shifting right means dividing by a power of 2
That means
- Code:
9 >> 2
= 9 / 4
= 2
Is it clear Or I should explain more?
BIT0102-Mohaimin- Programmer
- Course(s) :
- BIT
Blood Group : B+
Posts : 415
Points : 715
Re: Java Tutorial: Painting in swing components, part 1
Thanks vai a lot for explaining this. But we haven't been taught in EEE course , because it was Zerine Madam

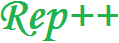
BIT0208-Shuvo- Global Moderator-RC
- Course(s) :
- BIT
Blood Group : B+
Posts : 87
Points : 124
Re: Java Tutorial: Painting in swing components, part 1
I assumed thatBIT0208-Shuvo wrote:Thanks vai a lot for explaining this. But we haven't been taught in EEE course , because it was Zerine Madam![]()
![]()

BIT0102-Mohaimin- Programmer
- Course(s) :
- BIT
Blood Group : B+
Posts : 415
Points : 715
Re: Java Tutorial: Painting in swing components, part 1
I am pleased and surprised to know it. I didn't know about it ago So interesting subject.............
topbdseo- Pre-Alpha Release
- Course(s) :
- N/A
Blood Group : NULL
Posts : 2
Points : 2

» Java Tutorial: Painting in swing components, part 2 (Interactive painting)
» Java Tutorial: Collection - Part 2 (List)
» Java Tutorial: Collection - Part 1 (Introduction)
» Java Swing: adding confirmation dialogue for closing Window in JFrame
» Java Tutorial: Using Two Java Reference Keywords, this and super
» Java Tutorial: Collection - Part 2 (List)
» Java Tutorial: Collection - Part 1 (Introduction)
» Java Swing: adding confirmation dialogue for closing Window in JFrame
» Java Tutorial: Using Two Java Reference Keywords, this and super
Page 1 of 1
Permissions in this forum:
You cannot reply to topics in this forum
|
|
» Cisco EHWIC SFP/GE WAN Card
» Huawei S1700-28GFR-4P-AC Price
» teach yourself C++ / Herbert Schildt Solutions
» teach yourself c by herbert schildt pdf
» ASA 5506X With Firepower ASA5506-K9
» New Trends in Deal Business
» PoE Power Allocation for WS-C2960S-24PS-L
» How to cure back pain